Albert Galan
prof. Erhan Mihail
Aplicație C#
Link-ul aplicației (WebGL):
https://atestatapp.albertgalan.ro/
Prima versiune: Crează un program pentru un concurs între consiliile școlare ale elevilor ale CNPR, CNSCM, CNISH și CNME, care să afișeze intrebări într-o ordine aleatorie, și să calculeze câte 2 puncte pentru fiecare răspuns corect.
A doua versiune: Modifică programul initial pentru a fi folosit în cadrul unor concursuri din cadrul școlii de vară de la CNISH, prin adăugarea unor noi moduri de joc.
Motivarea temei alese
Am ales aceasă temă pentru atestat deoarece a trebuit să învăț un limbaj nou de programare (C#), într-un timp record (am avut la dispoziție doar 2 săptămâni pentru a face prima versiune a programului, pentru modificarea ulterioară am avut însă 3 zile).
De asemenea, a trebuit să mă obișnuiesc cu mediul de programare Unity foarte repede, să învăț să înregistrez animații, să creez legături între cod și elementele grafice, și chiar să caut în tot codul o eroare (am scris “Team1Score” în loc de “Team1score“, primul fiind un element de tip text, al doilea fiind o variabilă de tip int).
Codul
Programul a fost realizat in cadrul Unity, fiind folosit ca și limbaj de programare C#.
Mai jos apare conținutul unora dintre cele mai importante fișiere din a doua versiune a aplicatiei, fiind explicate pe larg:
GameManager.cs
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.IO;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
using UnityEngine.Animations;
using TMPro;
public class GameManager : MonoBehaviour
{
public Question[] CNPRQuestions;
public Question[] CNPRFinalQuestions;
public Question[] CNSCMQuestions;
public Question[] CNSCMFinalQuestions;
public Question[] CNMEQuestions;
public Question[] CNMEFinalQuestions;
public Question[] CNISHQuestions;
public Question[] CNISHFinalQuestions;
public Question[] questions;
public Question[] NSCQuestions;
public Question[] PBQuestions;
private static List<Question> unansweredQuestions;
private Question currentQuestion;
private int AnswerTeam1;
private int AnswerTeam2;
[SerializeField] private Text questionText;
[SerializeField] private Text answer1;
[SerializeField] private Text answer2;
[SerializeField] private Text answer3;
[SerializeField] private Text answer4;
[SerializeField] private float timeBetweenQuestions = 5f;
[SerializeField] private Text Team1Name;
[SerializeField] private Text Team1Score;
[SerializeField] private Text Team2Name;
[SerializeField] private Text Team2Score;
private Animator anim;
[SerializeField] private Animator CheckMark;
[SerializeField] private Animator Checkmark1;
[SerializeField] private Animator Checkmark2;
[SerializeField] private Animator Checkmark3;
[SerializeField] private Animator Checkmark4;
// [SerializeField] private Animator NSCB1,NSCB2,NSCB3,NSCB4,NSCB5,NSCB6,NSCB7,NSCB8,NSCB9,NSCB10,NSCB11,NSCB12,NSCB13,NSCB14,NSCB15,NSCB16,NSCB18,NSCB19,NSCB20,NSCB21,NSCB22,NSCB23,NSCB24,NSCB25;
private string fileName = "Data.txt", myFilePath = Application.dataPath + "/Data/" + "Data.txt";
private int round, CNPRscore, CNSCMscore, CNMEscore, CNISHscore, CNPRfscore, CNSCMfscore, CNMEfscore, CNISHfscore;
private int Team1score, Team2score;
private static int AnsweredQuestionNumber = 0;
private int gamemode;
private int NSCscoreteam1, NSCscoreteam2, NSCQuestionNR, NSCQuestionScore;
private int PBscoreteam1, PBscoreteam2;
//CNISH
//NavigheazaSiCastigi
private int CNISHscoreteam1, CNISHscoreteam2;
void Start()
{
// if(unansweredQuestions == null || unansweredQuestions.Count == 0)
/*
gamemode = 1 Pe bune cu liceele
gamemode = 2 Navigheaza si castigi
gamemode = 3 Pe bune CNISH
*/
Load();
if (gamemode == 1) gamemode1();
if (gamemode == 2) gamemode2();
if (gamemode == 3) gamemode3();
}
void gamemode1()
{
if (round == 1)
{
Team1Name.text = "CNPR";
Team1score = CNPRscore;
Team2Name.text = "CNISH";
Team2score = CNISHscore;
questions = CNMEQuestions;
}
if (round == 2)
{
Team1Name.text = "CNSCM";
Team1score = CNSCMscore;
Team2Name.text = "CNME";
Team2score = CNMEscore;
questions = CNPRQuestions;
}
if (round == 3)
{
Team1Name.text = "CNPR";
Team1score = CNPRscore;
Team2Name.text = "CNSCM";
Team2score = CNSCMscore;
questions = CNISHQuestions;
}
if (round == 4)
{
Team1Name.text = "CNISH";
Team1score = CNISHscore;
Team2Name.text = "CNME";
Team2score = CNMEscore;
questions = CNSCMQuestions;
}
if (round == 5)
{
Team1Name.text = "CNPR";
Team1score = CNPRfscore;
Team2Name.text = "CNISH";
Team2score = CNISHfscore;
questions = CNSCMFinalQuestions.Concat(CNMEFinalQuestions).ToArray();
}
if (round == 6)
{
Team1Name.text = "CNSCM";
Team1score = CNSCMfscore;
Team2Name.text = "CNME";
Team2score = CNMEfscore;
questions = CNPRFinalQuestions.Concat(CNISHFinalQuestions).ToArray();
}
if (round == 7)
{
Team1Name.text = "CNPR";
Team1score = CNPRfscore;
Team2Name.text = "CNSCM";
Team2score = CNSCMfscore;
questions = CNMEFinalQuestions.Concat(CNISHFinalQuestions).ToArray();
}
if (round == 8)
{
Team1Name.text = "CNISH";
Team1score = CNISHfscore;
Team2Name.text = "CNME";
Team2score = CNMEfscore;
questions = CNSCMFinalQuestions.Concat(CNPRFinalQuestions).ToArray();
}
if (round == 9)
{
Team1Name.text = "CNPR";
Team1score = CNPRfscore;
Team2Name.text = "CNME";
Team2score = CNMEfscore;
questions = CNSCMFinalQuestions.Concat(CNISHFinalQuestions).ToArray();
}
if (round == 10)
{
Team1Name.text = "CNISH";
Team1score = CNISHfscore;
Team2Name.text = "CNSCM";
Team2score = CNMEfscore;
questions = CNPRFinalQuestions.Concat(CNMEFinalQuestions).ToArray();
}
Team1Score.text = "Score: " + Team1score;
Team2Score.text = "Score: " + Team2score;
Debug.Log("Round " + round);
//if (unansweredQuestions == null)
if (AnsweredQuestionNumber % 5 == 0)
{
Score1.scoreValue = Team1score;
Score2.scoreValue = Team2score;
unansweredQuestions = questions.ToList<Question>();
}
while (unansweredQuestions.Count > 5)
{
int randomQuestionNr = Random.Range(0, unansweredQuestions.Count);
unansweredQuestions.RemoveAt(randomQuestionNr);
}
anim = GetComponent<Animator>();
SetCurrentQuestion();
Debug.Log(currentQuestion.question + " " + currentQuestion.answer1 + " " + currentQuestion.answer2 + " " + currentQuestion.answer3 + " " + currentQuestion.answer4 + " answer " + currentQuestion.correctanswer);
}
void gamemode2()
{
Team1Name.text = "Team 1";
Team1score = NSCscoreteam1;
Team1Score.text = "Score: " + NSCscoreteam1;
Team2Name.text = "Team 2";
Team2score = NSCscoreteam2;
Team2Score.text = "Score: " + NSCscoreteam2;
questions = NSCQuestions;
//SetCurrentQuestion
currentQuestion = NSCQuestions[NSCQuestionNR];
NSCQuestionScore = ((NSCQuestionNR + 1) % 5) * 100;
if (NSCQuestionScore == 0) NSCQuestionScore = 500;
questionText.text = currentQuestion.question;
answer1.text = "1. " + currentQuestion.answer1;
answer2.text = "2. " + currentQuestion.answer2;
answer3.text = "3. " + currentQuestion.answer3;
answer4.text = "4. " + currentQuestion.answer4;
anim = GetComponent<Animator>();
Debug.Log(currentQuestion.question + " " + currentQuestion.answer1 + " " + currentQuestion.answer2 + " " + currentQuestion.answer3 + " " + currentQuestion.answer4 + " answer " + currentQuestion.correctanswer);
Debug.Log("NSCQuestionScore = " + NSCQuestionScore);
}
void gamemode3()
{
Team1Name.text = "Team 1";
Team1score = PBscoreteam1;
Team1Score.text = "Score: " + PBscoreteam1;
Team2Name.text = "Team 2";
Team2score = PBscoreteam2;
Team2Score.text = "Score: " + PBscoreteam2;
questions = PBQuestions;
if (unansweredQuestions == null)
{
Score1.scoreValue = Team1score;
Score2.scoreValue = Team2score;
unansweredQuestions = questions.ToList<Question>();
Debug.Log("unansweredQuestions = questions.ToList<Question>()");
}
anim = GetComponent<Animator>();
SetCurrentQuestion();
Debug.Log(currentQuestion.question + " " + currentQuestion.answer1 + " " + currentQuestion.answer2 + " " + currentQuestion.answer3 + " " + currentQuestion.answer4 + " answer " + currentQuestion.correctanswer);
}
private void Quit()
{
Application.Quit();
}
private void Save()
{
PlayerPrefs.SetInt("round", round);
PlayerPrefs.SetInt("CNPRscore", CNPRscore);
PlayerPrefs.SetInt("CNSCMscore", CNSCMscore);
PlayerPrefs.SetInt("CNMEscore", CNMEscore);
PlayerPrefs.SetInt("CNISHscore", CNISHscore);
PlayerPrefs.SetInt("CNPRfscore", CNPRfscore);
PlayerPrefs.SetInt("CNSCMfscore", CNSCMfscore);
PlayerPrefs.SetInt("CNMEfscore", CNMEfscore);
PlayerPrefs.SetInt("CNISHfscore", CNISHfscore);
PlayerPrefs.SetInt("NSCscoreteam1", NSCscoreteam1);
PlayerPrefs.SetInt("NSCscoreteam2", NSCscoreteam2);
PlayerPrefs.SetInt("NSCQuestionNR", NSCQuestionNR);
PlayerPrefs.SetInt("PBscoreteam1", PBscoreteam1);
PlayerPrefs.SetInt("PBscoreteam2", PBscoreteam2);
PlayerPrefs.Save();
Debug.Log("Saved");
}
private void Load()
{
int gamemode1 = PlayerPrefs.GetInt("gamemode");
int round1 = PlayerPrefs.GetInt("round");
int CNPRscore1 = PlayerPrefs.GetInt("CNPRscore", 0);
int CNSCMscore1 = PlayerPrefs.GetInt("CNSCMscore", 0);
int CNMEscore1 = PlayerPrefs.GetInt("CNMEscore", 0);
int CNISHscore1 = PlayerPrefs.GetInt("CNISHscore", 0);
int CNPRfscore1 = PlayerPrefs.GetInt("CNPRfscore", 0);
int CNSCMfscore1 = PlayerPrefs.GetInt("CNSCMfscore", 0);
int CNMEfscore1 = PlayerPrefs.GetInt("CNMEfscore", 0);
int CNISHfscore1 = PlayerPrefs.GetInt("CNISHfscore", 0);
int NSCscoreteam11 = PlayerPrefs.GetInt("NSCscoreteam1", 0);
int NSCscoreteam21 = PlayerPrefs.GetInt("NSCscoreteam2", 0);
int NSCQuestionNR1 = PlayerPrefs.GetInt("NSCQuestionNR", 0);
int PBscoreteam11 = PlayerPrefs.GetInt("PBscoreteam1", 0);
int PBscoreteam21 = PlayerPrefs.GetInt("PBscoreteam2", 0);
gamemode = gamemode1;
round = round1;
CNPRscore = CNPRscore1;
CNSCMscore = CNSCMscore1;
CNMEscore = CNMEscore1;
CNISHscore = CNISHscore1;
CNPRfscore = CNPRfscore1;
CNSCMfscore = CNSCMfscore1;
CNMEfscore = CNMEfscore1;
CNISHfscore = CNISHfscore1;
NSCscoreteam1 = NSCscoreteam11;
NSCscoreteam2 = NSCscoreteam21;
NSCQuestionNR = NSCQuestionNR1;
PBscoreteam1 = PBscoreteam11;
PBscoreteam2 = PBscoreteam21;
Debug.Log("Loaded");
}
/*
private void Save()
{
PlayerPrefs.SetInt("round", round);
PlayerPrefs.SetInt("CNPRscore", CNPRscore);
PlayerPrefs.SetInt("CNSCMscore", CNSCMscore);
PlayerPrefs.SetInt("CNMEscore", CNMEscore);
PlayerPrefs.SetInt("CNISHscore", CNISHscore);
PlayerPrefs.SetInt("CNPRfscore", CNPRfscore);
PlayerPrefs.SetInt("CNSCMfscore", CNSCMfscore);
PlayerPrefs.SetInt("CNMEfscore", CNMEfscore);
PlayerPrefs.SetInt("CNISHfscore", CNISHfscore);
PlayerPrefs.SetInt("CNISHscoreteam1", CNISHscoreteam1);
PlayerPrefs.SetInt("CNISHscoreteam2", CNISHscoreteam2);
PlayerPrefs.Save();
Debug.Log("Saved");
}
private void Load()
{
int gamemode1 = PlayerPrefs.GetInt("gamemode");
int round1 = PlayerPrefs.GetInt("round");
int CNPRscore1 = PlayerPrefs.GetInt("CNPRscore", 0);
int CNSCMscore1 = PlayerPrefs.GetInt("CNSCMscore", 0);
int CNMEscore1 = PlayerPrefs.GetInt("CNMEscore", 0);
int CNISHscore1 = PlayerPrefs.GetInt("CNISHscore", 0);
int CNPRfscore1 = PlayerPrefs.GetInt("CNPRfscore", 0);
int CNSCMfscore1 = PlayerPrefs.GetInt("CNSCMfscore", 0);
int CNMEfscore1 = PlayerPrefs.GetInt("CNMEfscore", 0);
int CNISHfscore1 = PlayerPrefs.GetInt("CNISHfscore", 0);
int CNISHscoreteam11 = PlayerPrefs.GetInt("CNISHscoreteam1", 0);
int CNISHscoreteam21 = PlayerPrefs.GetInt("CNISHscoreteam2", 0);
gamemode = gamemode1;
round = round1;
CNPRscore = CNPRscore1;
CNSCMscore = CNSCMscore1;
CNMEscore = CNMEscore1;
CNISHscore = CNISHscore1;
CNPRfscore = CNPRfscore1;
CNSCMfscore = CNSCMfscore1;
CNMEfscore = CNMEfscore1;
CNISHfscore = CNISHfscore1;
CNISHscoreteam1 = CNISHscoreteam11;
CNISHscoreteam2 = CNISHscoreteam21;
Debug.Log("Loaded");
}
*/
void SetCurrentQuestion()
{
int randomQuestionNr = Random.Range(0, unansweredQuestions.Count);
currentQuestion = unansweredQuestions[randomQuestionNr];
questionText.text = (AnsweredQuestionNumber % 5 + 1) + ". " + currentQuestion.question;
answer1.text = "1. " + currentQuestion.answer1;
answer2.text = "2. " + currentQuestion.answer2;
answer3.text = "3. " + currentQuestion.answer3;
answer4.text = "4. " + currentQuestion.answer4;
// unansweredQuestions.RemoveAt(randomQuestionNr);
}
IEnumerator TransitionToNextQuestion()
{
if (round == 1)
{
CNPRscore = Team1score;
CNISHscore = Team2score;
}
if (round == 2)
{
CNSCMscore = Team1score;
CNMEscore = Team2score;
}
if (round == 3)
{
CNPRscore = Team1score;
CNSCMscore = Team2score;
}
if (round == 4)
{
CNISHscore = Team1score;
CNMEscore = Team2score;
}
if (round == 5)
{
CNPRfscore = Team1score;
CNISHfscore = Team2score;
}
if (round == 6)
{
CNSCMfscore = Team1score;
CNMEfscore = Team2score;
}
if (round == 7)
{
CNPRfscore = Team1score;
CNSCMfscore = Team2score;
}
if (round == 8)
{
CNISHfscore = Team1score;
CNMEfscore = Team2score;
}
if (round == 9)
{
CNPRfscore = Team1score;
CNMEfscore = Team2score;
}
if (round == 10)
{
CNISHfscore = Team1score;
CNMEfscore = Team2score;
}
if (gamemode == 2)
{
NSCscoreteam1 = Team1score;
NSCscoreteam2 = Team2score;
}
if (gamemode == 3)
{
PBscoreteam1 = Team1score;
PBscoreteam2 = Team2score;
}
AnsweredQuestionNumber++;
Debug.Log("Round " + round);
Save();
if (gamemode != 2)
{
if (unansweredQuestions.Count > 1)
unansweredQuestions.Remove(currentQuestion);
yield return new WaitForSeconds(timeBetweenQuestions);
if (AnsweredQuestionNumber % 5 == 0)
SceneManager.LoadScene("Main");
else SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
else
{
yield return new WaitForSeconds(timeBetweenQuestions);
SceneManager.LoadScene("NavigheziSiCastigi");
}
/*
if(AnsweredQuestionNumber < 5)
if (unansweredQuestions.Count > 0)
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
else SceneManager.LoadScene("Main");
*/
}
public void Team1SelectAnswer1()
{
if (currentQuestion.correctanswer == 1)
{
Debug.Log("Correct");
if (gamemode == 1)
Team1score += 2;
if (gamemode == 2)
Team1score = Team1score + NSCQuestionScore;
if(gamemode == 3)
Team1score ++;
Score1.scoreValue = Team1score;
}
else
{
Debug.Log("Wrong");
}
// FalseButton.Play(TrueAnimation, 0, 0.1f);
}
public void Team1SelectAnswer2()
{
if (currentQuestion.correctanswer == 2)
{
Debug.Log("Correct");
if (gamemode == 1)
Team1score += 2;
if (gamemode == 2)
Team1score = Team1score + NSCQuestionScore;
if(gamemode == 3)
Team1score ++;
Score1.scoreValue = Team1score;
}
else
{
Debug.Log("Wrong");
}
}
public void Team1SelectAnswer3()
{
if (currentQuestion.correctanswer == 3)
{
Debug.Log("Correct");
if (gamemode == 1)
Team1score += 2;
if (gamemode == 2)
Team1score = Team1score + NSCQuestionScore;
if(gamemode == 3)
Team1score ++;
Score1.scoreValue = Team1score;
}
else
{
Debug.Log("Wrong");
}
}
public void Team1SelectAnswer4()
{
if (currentQuestion.correctanswer == 4)
{
Debug.Log("Correct");
if (gamemode == 1)
Team1score += 2;
if (gamemode == 2)
Team1score = Team1score + NSCQuestionScore;
if(gamemode == 3)
Team1score ++;
Score1.scoreValue = Team1score;
}
else
{
Debug.Log("Wrong");
}
}
public void Team2SelectAnswer1()
{
if (currentQuestion.correctanswer == 1)
{
Debug.Log("Correct");
if (gamemode == 1)
Team2score += 2;
if (gamemode == 2)
Team2score = Team2score + NSCQuestionScore;
if(gamemode == 3)
Team2score ++;
Score2.scoreValue = Team2score;
}
else
{
Debug.Log("Wrong");
}
// FalseButton.Play(TrueAnimation, 0, 0.1f);
}
public void Team2SelectAnswer2()
{
if (currentQuestion.correctanswer == 2)
{
Debug.Log("Correct");
if (gamemode == 1)
Team2score += 2;
if (gamemode == 2)
Team2score = Team2score + NSCQuestionScore;
if(gamemode == 3)
Team2score ++;
Score2.scoreValue = Team2score;
}
else
{
Debug.Log("Wrong");
}
}
public void Team2SelectAnswer3()
{
if (currentQuestion.correctanswer == 3)
{
Debug.Log("Correct");
if (gamemode == 1)
Team2score += 2;
if (gamemode == 2)
Team2score = Team2score + NSCQuestionScore;
if(gamemode == 3)
Team2score ++;
Score2.scoreValue = Team2score;
}
else
{
Debug.Log("Wrong");
}
}
public void Team2SelectAnswer4()
{
if (currentQuestion.correctanswer == 4)
{
Debug.Log("Correct");
if (gamemode == 1)
Team2score += 2;
if (gamemode == 2)
Team2score = Team2score + NSCQuestionScore;
if(gamemode == 3)
Team2score ++;
Score2.scoreValue = Team2score;
}
else
{
Debug.Log("Wrong");
}
}
public void UserSelectAnswer1()
{
if (currentQuestion.correctanswer == 1)
{
Score1.scoreValue += 2;
Debug.Log("Correct");
}
else
{
Debug.Log("Wrong");
}
//CorrectAnswerAnimation(currentQuestion.correctanswer);
//CorrectAnimation(currentQuestion.correctanswer);
//CheckMark.Play("Answer1", 0, 10f);
//anim.Play("Answer1", 0, 0.25f);
// FalseButton.Play(TrueAnimation, 0, 0.1f);
//StartCoroutine(TransitionToNextQuestion());
}
public void UserSelectAnswer2()
{
if (currentQuestion.correctanswer == 2)
{
Debug.Log("Correct");
Score1.scoreValue += 2;
}
else
{
Debug.Log("Wrong");
}
}
public void UserSelectAnswer3()
{
if (currentQuestion.correctanswer == 3)
{
Debug.Log("Correct");
Score1.scoreValue += 2;
}
else
{
Debug.Log("Wrong");
}
}
public void UserSelectAnswer4()
{
if (currentQuestion.correctanswer == 4)
{
Debug.Log("Correct");
Score1.scoreValue += 2;
}
else
{
Debug.Log("Wrong");
}
}
public void CorrectAnimation(int correctanswer)
{
string answer = "Answer";
answer = answer + correctanswer;
CheckMark.Play(answer, 0, 10f);
}
public void CorrectAnswerAnimation(int PlayerAnswer)
{
if (PlayerAnswer == 1)
Checkmark1.Play("Answer1Correct", 0, 10f);
if (PlayerAnswer == 2)
Checkmark2.Play("Answer2Correct", 0, 10f);
if (PlayerAnswer == 3)
Checkmark3.Play("Answer3Correct", 0, 10f);
if (PlayerAnswer == 4)
Checkmark4.Play("Answer4Correct", 0, 10f);
}
public void NextQuestion()
{
CorrectAnswerAnimation(currentQuestion.correctanswer);
StartCoroutine(TransitionToNextQuestion());
}
}
GameManager.cs e funția principală a aplicatiei. Ea face aproape tot, în functie de modul de joc și de rundă selectează și afișează întrebările, verifică răspunsurile și acordă punctajele corespunzătoare și reîncarcă scena cu noua întrebare, la urmă revenind la scena precedentă.
Question.cs
[System.Serializable]
public class Question
{
public string question;
public string answer1;
public string answer2;
public string answer3;
public string answer4;
public int correctanswer;
}
Question.cs e echivalentul unui struct din C++, declarând un nou obiect de tip Question, compus din 5 string-uri si un întreg.
ChangeSceneWithButton.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class ChangeSceneWithButton : MonoBehaviour
{
public void LoadScene(string sceneName)
{
SceneManager.LoadScene(sceneName);
}
}
ChangeSceneWithButton e o funcție care permite navigarea între scene, fiind executată la interacția utilizatorului cu un buton căruia i s-a adăugat această funcție si care a primit ca parametru numele scenei care trebuie încărcată.
SaveHandler.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SaveHandler : MonoBehaviour
{
public void WipeSave()
{
PlayerPrefs.DeleteAll();
Debug.Log("Save wiped");
}
}
SaveHandler.cs avea inițial rolul de a salva permanent scorurile obtinute de jucători, dar și de a le reîncărca. Aceste funcții au fost mutate in GameManager.cs pentru a putea fi executate mai ușor. Acum, singura funcție rămasă este cea de WipeSave(), care, apelată de un buton, sterge toate datele salvate, resetând jocul.
SceneControl.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class SceneControl : MonoBehaviour
{
int round, ok;
int gamemode;
public void LoadScene(string sceneName)
{
if (sceneName == "CNPR-CNISH")
round = 1;
if (sceneName == "CNSCM-CNME")
round = 2;
if (sceneName == "CNPR-CNSCM")
round = 3;
if (sceneName == "CNISH-CNME")
round = 4;
if (sceneName == "CNPR-CNISH-F")
round = 5;
if (sceneName == "CNSCM-CNME-F")
round = 6;
if (sceneName == "CNPR-CNSCM-F")
round = 7;
if (sceneName == "CNISH-CNME-F")
round = 8;
if (sceneName == "CNPR-CNME-F")
round = 9;
if (sceneName == "CNISH-CNSCM-F")
round = 10;
ok = 1;
PlayerPrefs.SetInt("ok", ok);
PlayerPrefs.SetInt("round", round);
SceneManager.LoadScene("test");
}
IEnumerator waiter(int time)
{
for(int z=0; z<time; z++)
{
Debug.Log("waiting");
yield return new WaitForSeconds(time);
Debug.Log("waited " + z + " seconds");
}
}
public void mainmenubutton()
{
int gamemode = PlayerPrefs.GetInt("gamemode");
if (gamemode == 1 )
SceneManager.LoadScene("Main");
if (gamemode == 2)
SceneManager.LoadScene("NavigheziSiCastigi");
if (gamemode == 3)
SceneManager.LoadScene("PeBuneCNISH");
}
public void scoreboardbutton()
{
int gamemode = PlayerPrefs.GetInt("gamemode");
if (gamemode == 1)
{
SceneManager.LoadScene("scoreboard");
Debug.Log("Scoreboard");
}
else
{
SceneManager.LoadScene("scoreboardCNISH");
if (gamemode == 2)
Debug.Log("ScoreboardNavigheaziSiCastigi");
else
Debug.Log("ScoreboardPeBuneCNISH");
}
}
public void backbutton()
{
int gamemode = PlayerPrefs.GetInt("gamemode");
if (gamemode == 1) SceneManager.LoadScene("Main");
if (gamemode == 2) SceneManager.LoadScene("NavigheziSiCastigi");
if (gamemode == 3) SceneManager.LoadScene("PeBuneCNISH");
}
public void LoadSceneByName(string sceneName)
{
//StartCoroutine(waiter(2));
SceneManager.LoadScene(sceneName);
}
public void SelectGameMode(int gamemode)
{
/*
gamemode = 1 Pe bune cu liceele
gamemode = 2 Navigheaza si castigi
gamemode = 3 Pe bune CNISH
*/
PlayerPrefs.SetInt("gamemode", gamemode);
Debug.Log("Gamemode = " + gamemode);
if (gamemode == 1) SceneManager.LoadScene("Main");
if (gamemode == 2) SceneManager.LoadScene("NavigheziSiCastigi");
if (gamemode == 3) SceneManager.LoadScene("PeBuneCNISH");
}
public void GameMode2SelectQuestion(int NSCQuestionNR)
{
NSCQuestionNR--;
PlayerPrefs.SetInt("NSCQuestionNR", NSCQuestionNR);
Debug.Log("Game mode 2 Question " + NSCQuestionNR);
SceneManager.LoadScene("Test");
}
/*
public void GameMode2SelectQuestionwithcolor(int NSCQuestionNR)
{
NSCQuestionNR--;
PlayerPrefs.SetInt("NSCQuestionNR", NSCQuestionNR);
Debug.Log("Game mode 2 Question " + NSCQuestionNR);
GetComponent<Image>().color = Color.red;
SceneManager.LoadScene("Test");
}
*/
public void Quit()
{
Application.Quit();
//this.Close();
}
}
SceneControl.cs are la bază ChangeSceneWithButton.cs, adăugând însă alte funcții, cum ar fi cea de a ajuta GameManager.cs să știe ce întrebări să încarce, scorurile pentru fiecare răspuns corect, dar și cele corespunzătoare butoanelor de “Back” și “Home”.
ScoreController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ScoreController : MonoBehaviour
{
[SerializeField] private Text CNPRscoreText;
[SerializeField] private Text CNSCMscoreText;
[SerializeField] private Text CNMEscoreText;
[SerializeField] private Text CNISHscoreText;
[SerializeField] private Text CNPRfscoreText;
[SerializeField] private Text CNSCMfscoreText;
[SerializeField] private Text CNMEfscoreText;
[SerializeField] private Text CNISHfscoreText;
[SerializeField] private Text Team1CNISHscoreText;
[SerializeField] private Text Team2CNISHscoreText;
[SerializeField] private Text GameModeText;
/*
[SerializeField] public InputField CNPR;
[SerializeField] public InputField CNPRf;
[SerializeField] public InputField CNSCM;
[SerializeField] public InputField CNSCMf;
[SerializeField] public InputField CNME;
[SerializeField] public InputField CNMEf;
[SerializeField] public InputField CNISH;
[SerializeField] public InputField CNISHf;
[SerializeField] public InputField PeBuneTeam1CNISH;
[SerializeField] public InputField PeBuneTeam2CNISH;
*/
private int round, CNPRscore, CNSCMscore, CNMEscore, CNISHscore, CNPRfscore, CNSCMfscore, CNMEfscore, CNISHfscore;
private int NSCscoreteam1, NSCscoreteam2, NSCQuestionNR;
private int PBscoreteam1, PBscoreteam2;
private int gamemode;
// Start is called before the first frame update
void Start()
{
Load();
CNPRscoreText.text = "Score: " + CNPRscore;
CNSCMscoreText.text = "Score: " + CNSCMscore;
CNMEscoreText.text = "Score: " + CNMEscore;
CNISHscoreText.text = "Score: " + CNISHscore;
CNPRfscoreText.text = "FScore: " + CNPRfscore;
CNSCMfscoreText.text = "FScore: " + CNSCMfscore;
CNMEfscoreText.text = "FScore: " + CNMEfscore;
CNISHfscoreText.text = "FScore: " + CNISHfscore;
if (gamemode == 1)
GameModeText.text = "Pe Bune cu liceele";
if (gamemode == 2)
{
Team1CNISHscoreText.text = "Score: " + NSCscoreteam1;
Team2CNISHscoreText.text = "Score: " + NSCscoreteam2;
GameModeText.text = "Navighezi si Castigi";
}
if (gamemode == 3)
{
Team1CNISHscoreText.text = "Score: " + PBscoreteam1;
Team2CNISHscoreText.text = "Score: " + PBscoreteam2;
GameModeText.text = "Pe Bune CNISH";
}
Debug.Log("Gamemode = " + gamemode);
}
// Update is called once per frame
void Update()
{
/*
Load();
CNPRscoreText.text = "Score: " + CNPRscore;
CNSCMscoreText.text = "Score: " + CNSCMscore;
CNMEscoreText.text = "Score: " + CNMEscore;
CNISHscoreText.text = "Score: " + CNISHscore;
CNPRfscoreText.text = "FScore: " + CNPRfscore;
CNSCMfscoreText.text = "FScore: " + CNSCMfscore;
CNMEfscoreText.text = "FScore: " + CNMEfscore;
CNISHfscoreText.text = "FScore: " + CNISHfscore;
Debug.Log(CNISHscoreteam1);
PeBuneTeam1CNISHscoreText.text = "Score: " + CNISHscoreteam1;
PeBuneTeam2CNISHscoreText.text = "Score: " + CNISHscoreteam2;
CNPRscore = System.Convert.ToInt32(CNPR.text);
CNSCMscore = System.Convert.ToInt32(CNSCM.text);
CNMEscore = System.Convert.ToInt32(CNME.text);
CNISHscore = System.Convert.ToInt32(CNISH.text);
CNPRfscore = System.Convert.ToInt32(CNPRf.text);
CNSCMfscore = System.Convert.ToInt32(CNSCMf.text);
CNMEfscore = System.Convert.ToInt32(CNMEf.text);
CNISHfscore = System.Convert.ToInt32(CNISHf.text);
Save();
*/
}
private void Save()
{
PlayerPrefs.SetInt("round", round);
PlayerPrefs.SetInt("CNPRscore", CNPRscore);
PlayerPrefs.SetInt("CNSCMscore", CNSCMscore);
PlayerPrefs.SetInt("CNMEscore", CNMEscore);
PlayerPrefs.SetInt("CNISHscore", CNISHscore);
PlayerPrefs.SetInt("CNPRfscore", CNPRfscore);
PlayerPrefs.SetInt("CNSCMfscore", CNSCMfscore);
PlayerPrefs.SetInt("CNMEfscore", CNMEfscore);
PlayerPrefs.SetInt("CNISHfscore", CNISHfscore);
PlayerPrefs.SetInt("NSCscoreteam1", NSCscoreteam1);
PlayerPrefs.SetInt("NSCscoreteam2", NSCscoreteam2);
PlayerPrefs.SetInt("NSCQuestionNR", NSCQuestionNR);
PlayerPrefs.SetInt("PBscoreteam1", PBscoreteam1);
PlayerPrefs.SetInt("PBscoreteam2", PBscoreteam2);
PlayerPrefs.Save();
Debug.Log("Saved");
}
private void Load()
{
int gamemode1 = PlayerPrefs.GetInt("gamemode");
int round1 = PlayerPrefs.GetInt("round");
int CNPRscore1 = PlayerPrefs.GetInt("CNPRscore", 0);
int CNSCMscore1 = PlayerPrefs.GetInt("CNSCMscore", 0);
int CNMEscore1 = PlayerPrefs.GetInt("CNMEscore", 0);
int CNISHscore1 = PlayerPrefs.GetInt("CNISHscore", 0);
int CNPRfscore1 = PlayerPrefs.GetInt("CNPRfscore", 0);
int CNSCMfscore1 = PlayerPrefs.GetInt("CNSCMfscore", 0);
int CNMEfscore1 = PlayerPrefs.GetInt("CNMEfscore", 0);
int CNISHfscore1 = PlayerPrefs.GetInt("CNISHfscore", 0);
int NSCscoreteam11 = PlayerPrefs.GetInt("NSCscoreteam1", 0);
int NSCscoreteam21 = PlayerPrefs.GetInt("NSCscoreteam2", 0);
int NSCQuestionNR1 = PlayerPrefs.GetInt("NSCQuestionNR", 0);
int PBscoreteam11 = PlayerPrefs.GetInt("PBscoreteam1", 0);
int PBscoreteam21 = PlayerPrefs.GetInt("PBscoreteam2", 0);
gamemode = gamemode1;
round = round1;
CNPRscore = CNPRscore1;
CNSCMscore = CNSCMscore1;
CNMEscore = CNMEscore1;
CNISHscore = CNISHscore1;
CNPRfscore = CNPRfscore1;
CNSCMfscore = CNSCMfscore1;
CNMEfscore = CNMEfscore1;
CNISHfscore = CNISHfscore1;
NSCscoreteam1 = NSCscoreteam11;
NSCscoreteam2 = NSCscoreteam21;
NSCQuestionNR = NSCQuestionNR1;
PBscoreteam1 = PBscoreteam11;
PBscoreteam2 = PBscoreteam21;
Debug.Log("Loaded");
}
}
ScoreController.cs are rolul de a afișa scorurile în scena “ManualScoreOverride”, functionalitatea de a modifica manual scorurile nefiind funcțională încă, referirile la aceasta fiind comentate pentru a nu cauza erori în timpul execuției.
Score1.cs && Score2.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Score1 : MonoBehaviour
{
public static int scoreValue = 0;
Text score;
// Start is called before the first frame update
void Start()
{
score = GetComponent<Text>();
score.text = "Score: " + scoreValue;
}
// Update is called once per frame
void Update()
{
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Score2 : MonoBehaviour
{
public static int scoreValue = 0;
Text score;
// Start is called before the first frame update
void Start()
{
score = GetComponent<Text>();
score.text = "Score: " + scoreValue;
}
// Update is called once per frame
void Update()
{
}
}
Aceste funții complementează GameManager.cs pentru afișarea scorurilor echipelor. Afișarea are loc doar la reîncărcarea scenei, eliminând posibilitatea copierii răspunsului de la echipa adversă.
Scenele
Scenele, localizate în folderul Scenes au fost realizate prin intermediul interfaței Unity, folosind elemente MaterialUI pentru aspectul mai elevat decât cele oferite de Unity.
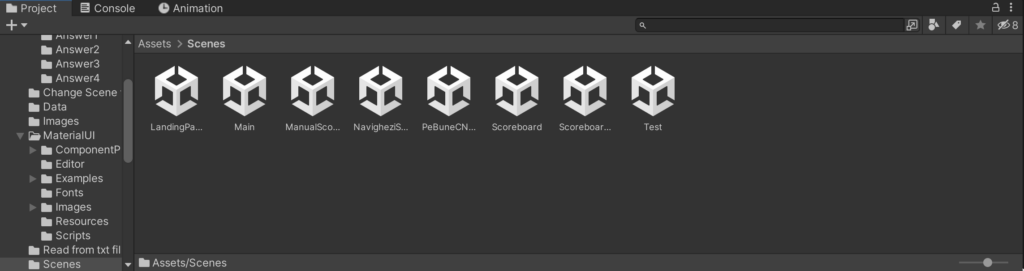
LandingPage
Această scenă este prima care se încarcă în momentul lansării în execuție, permițând selectarea modului de joc, dar și resetarea datelor sesiunilor anterioare.
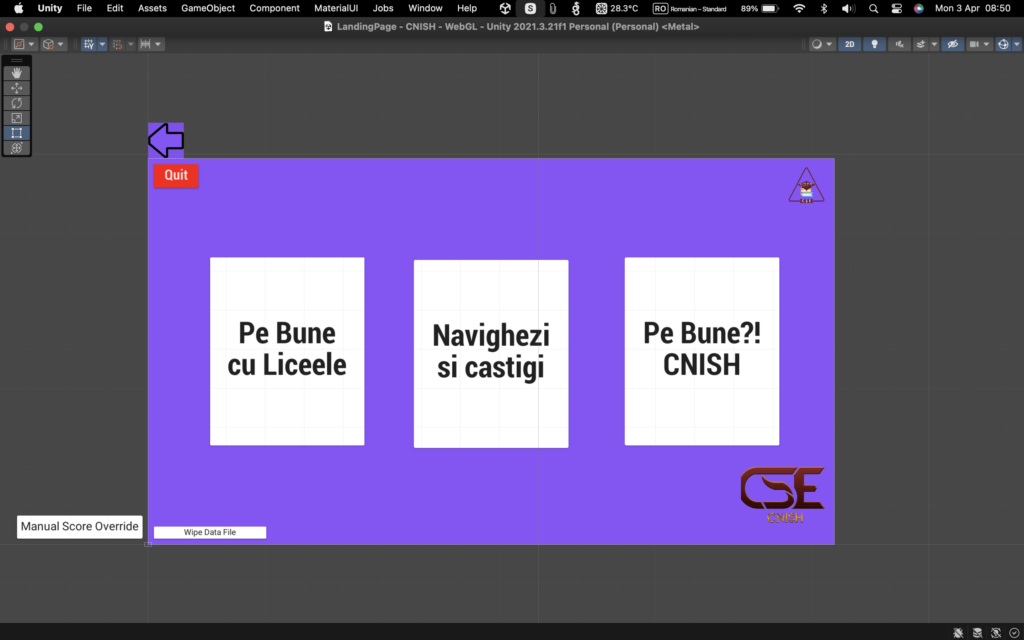
Main
Aceasta este scena principală pentru prima versiune a aplicației, în a doua devenind primul mod de joc, concursul între licee.
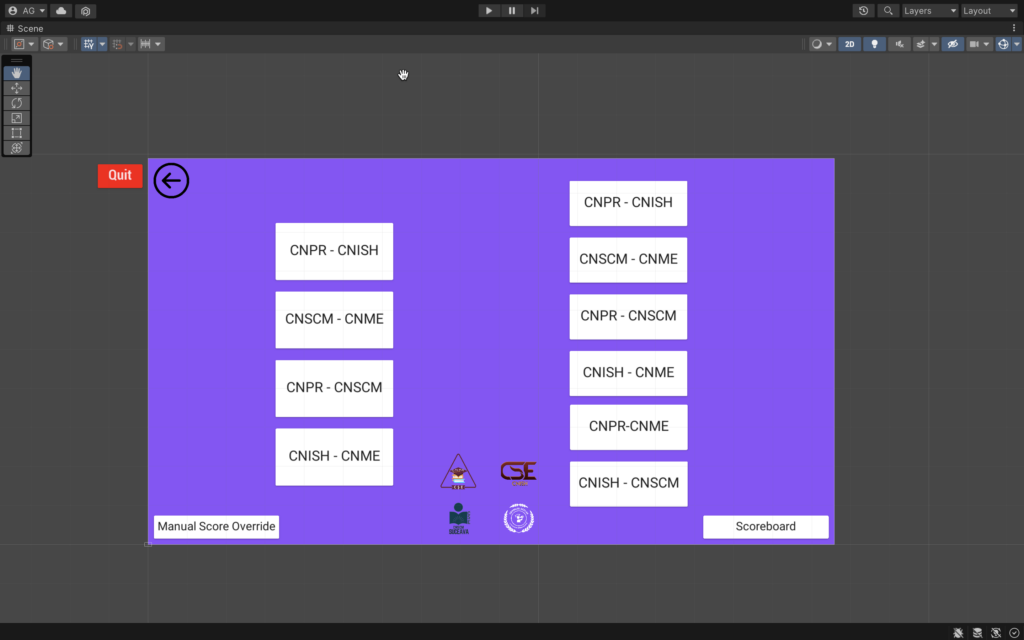
NavigheziSiCastigi
Aceasta este scena principală pentru al doilea mod de joc, “Navighezi Și Câștigi”, adăugată la cererea celor de la CNISH.
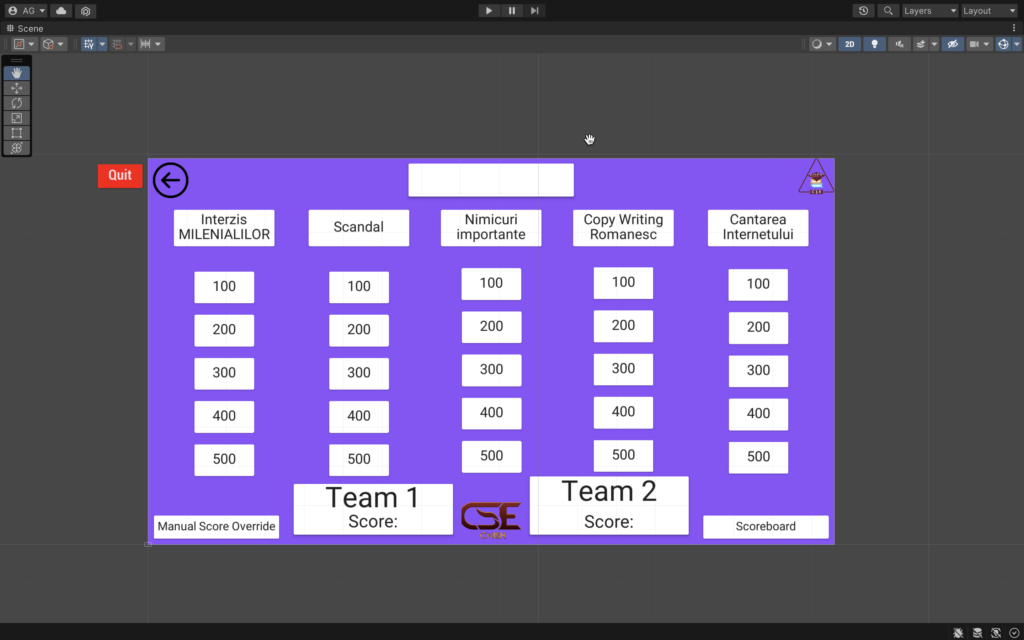
PeBuneCNISH
Aceasta este scena de pornire pentru al treilea mod de joc, de asemenea pentru cei de la CNISH.
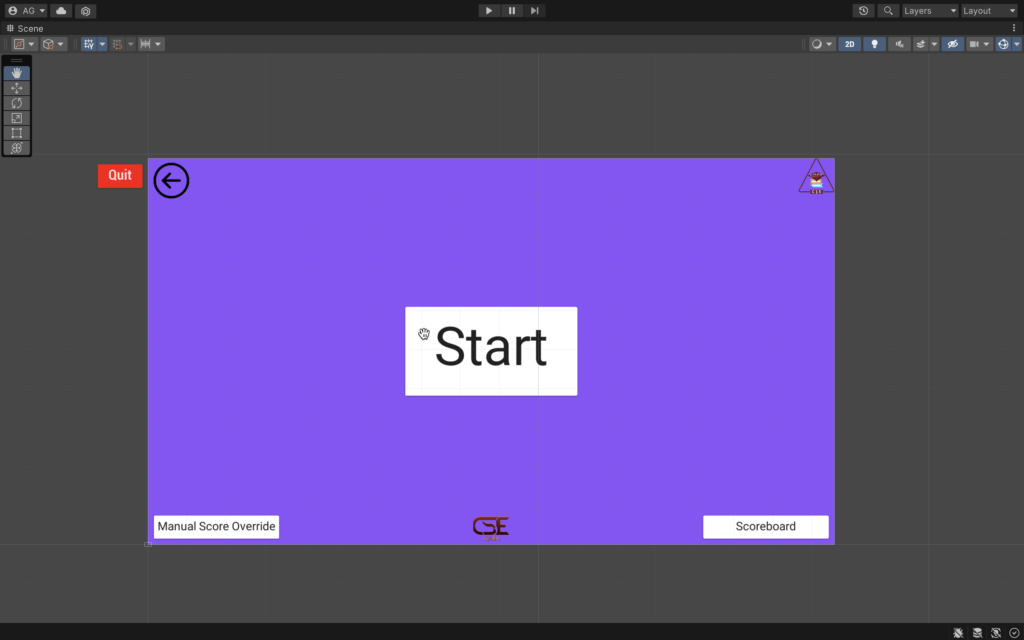
Scoreboard
Acesta este podiumul pentru primul mod de joc, afișând scorurile obținute de echipele liceelor.
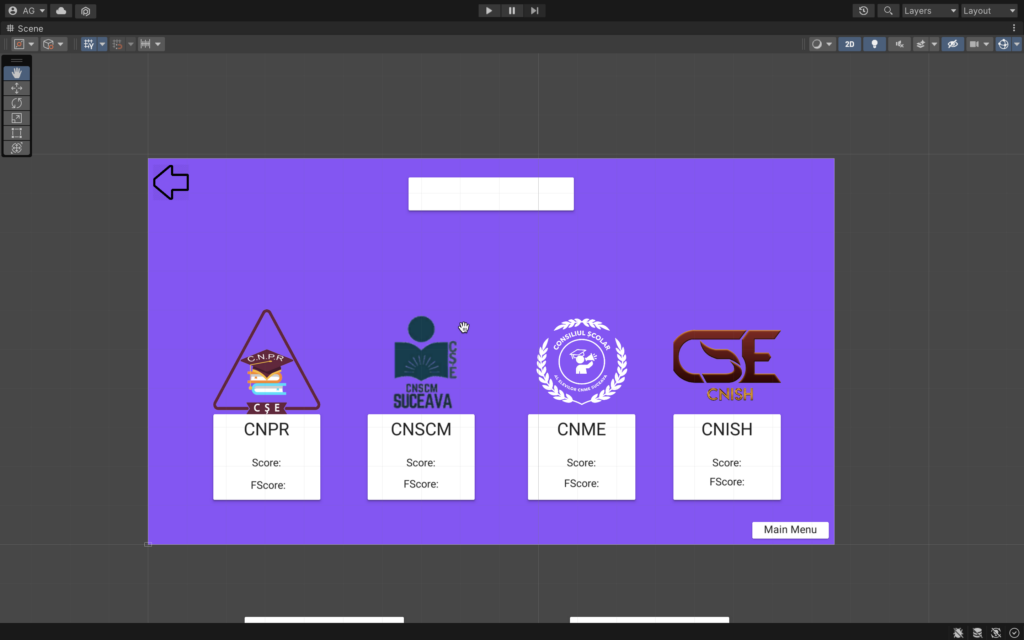
ScoreboardCNISH
Acesta este poiumul pentru al doilea mod de joc, cât și pentru al treilea.
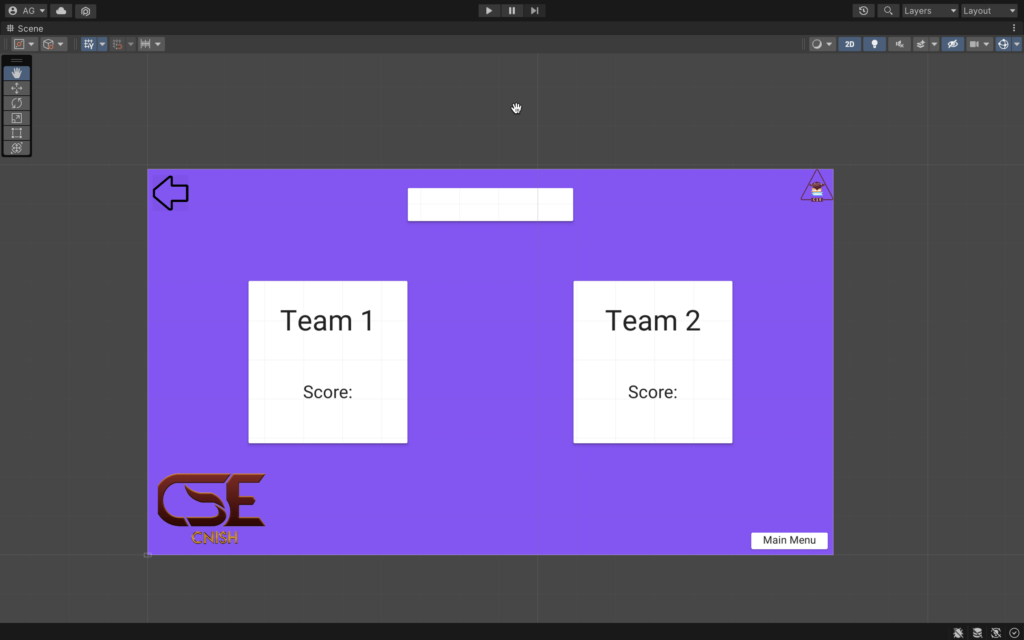
Test
“Test” este cea mai importantă scenă, fiind cea care afișează întrebările.
(A fost inițial un test pentru funcționalitatea GameManager.cs, de unde și numele, apoi fiind modificat aspectul, numele fiind lăsat la fel pentru a nu strica legăturile între scene)

Folderele proiectului
Folderul Assets conține fișierele programului, cum ar fi scripturile C# mentionate mai sus, pozele, scenele, dar și animațiile necesare funcționării programului.
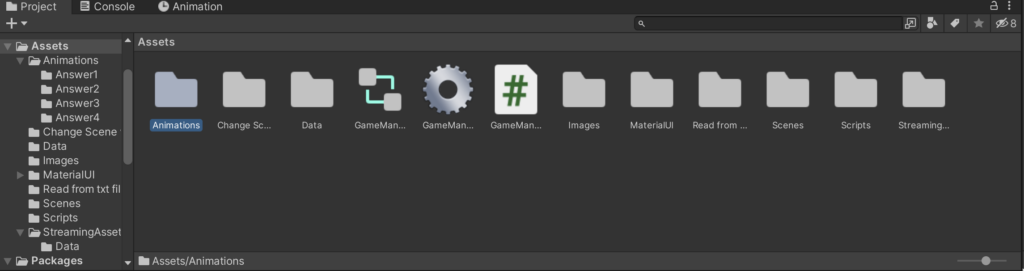
Scripturile sunt localizate în folderul Scripts, cele mai importante fiind prezentate mai sus.
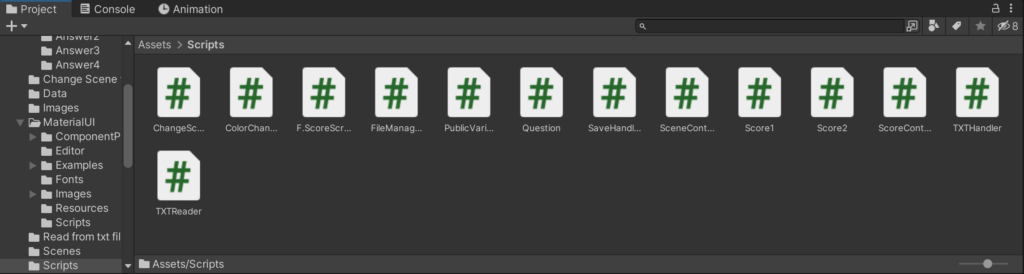
Animațiile evidențiază răspunsul corect, fiind apelate de către GameManager.cs.
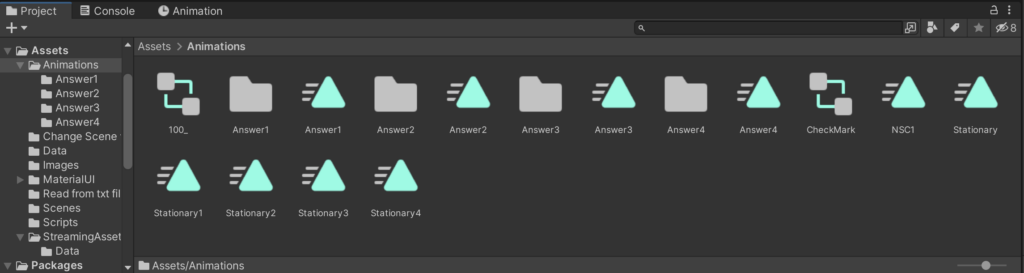
În Images sunt localizate siglele liceelor, dar și bifele pentru răspunsurile corecte și icon-ul butonului de “back”.
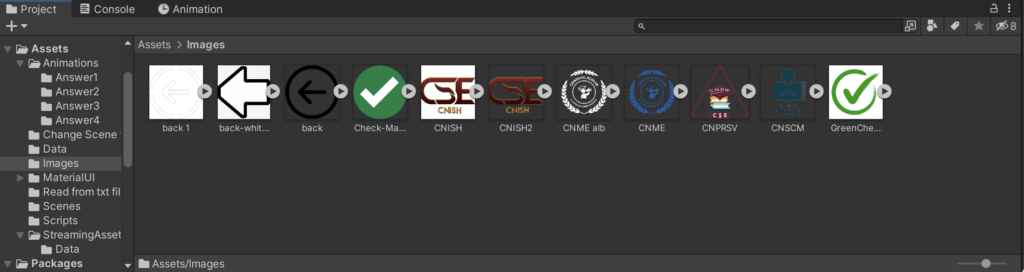
Game Objects
Acestea sunt în general butoanele, câmpurile de text, panourile, și altele.
În scene, avem si obiecte care nu apar pe ecran, rolul acestora fiind de a executa scripturile și de a controla elementele vizuale (câmpurile de text, animațiile, butoanele), cum ar fi:
GameManager
Acest obiect execută scriptul GameManager.cs.
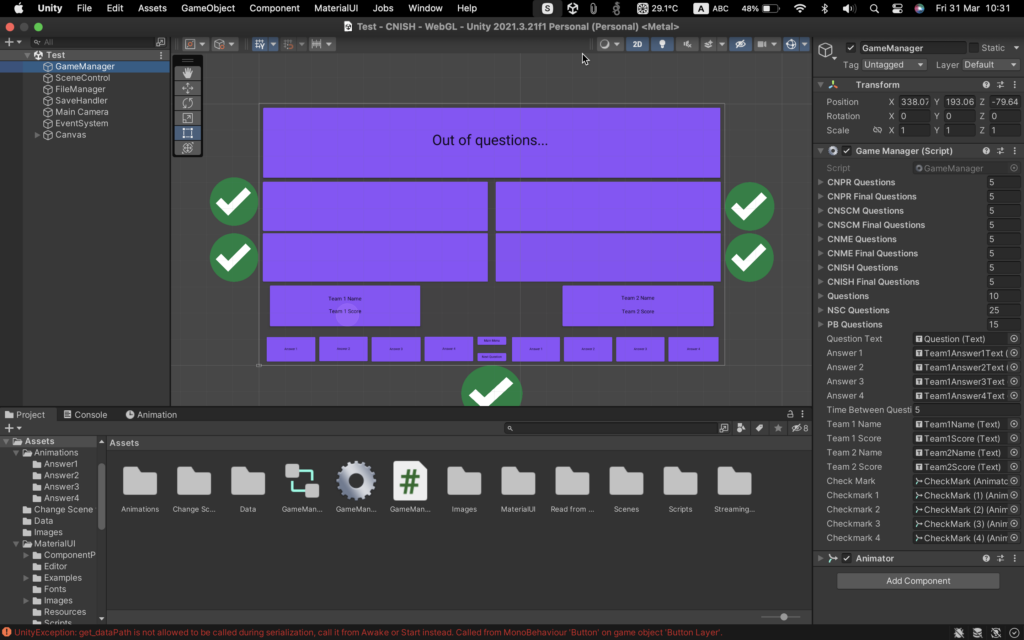
De asemenea, datorită declarării cu “SerializeField”
[SerializeField] private float timeBetweenQuestions = 5f;
a variabilelor, întrebările se adaugă din interfața grafică Unity, acestea fiind mult mai ușor de adăugat sau eliminat, numărul acestora fiind furnizat tot de interfață.
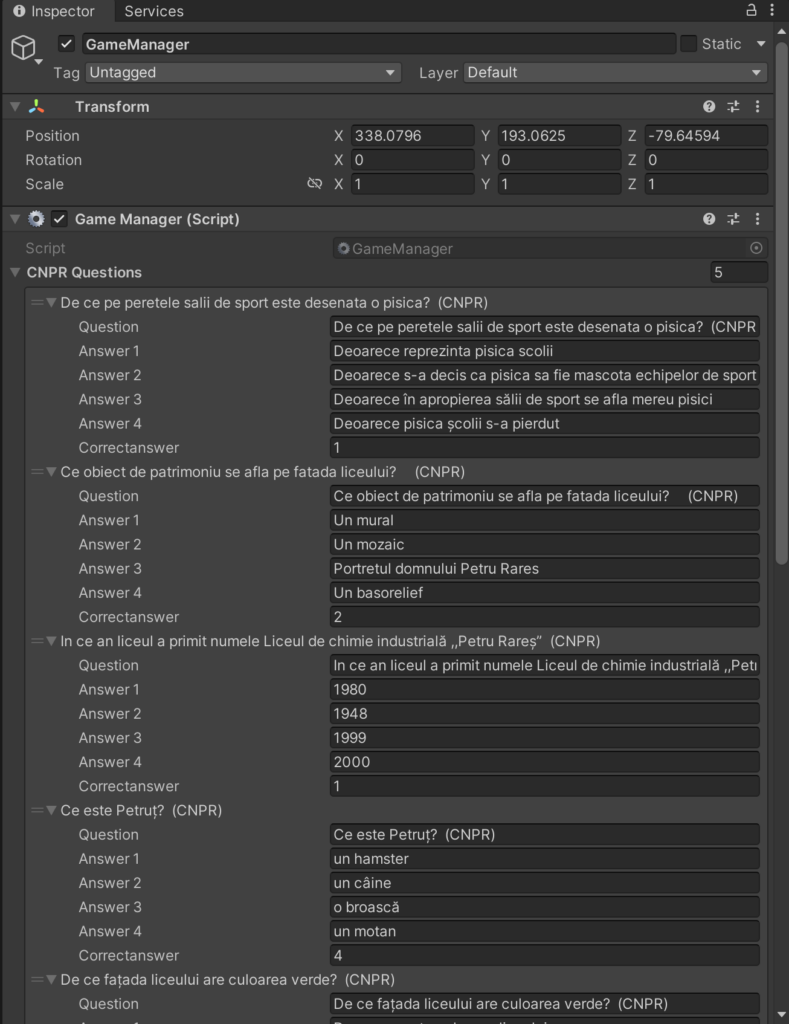
Tot datorită acestei declarări, de data aceasta pentru obiectele jocului
[SerializeField] private Text questionText;
ne este permis să legăm codul de elementele grafice, apelând animații, schimbând textul de pe panouri, și multe altele.
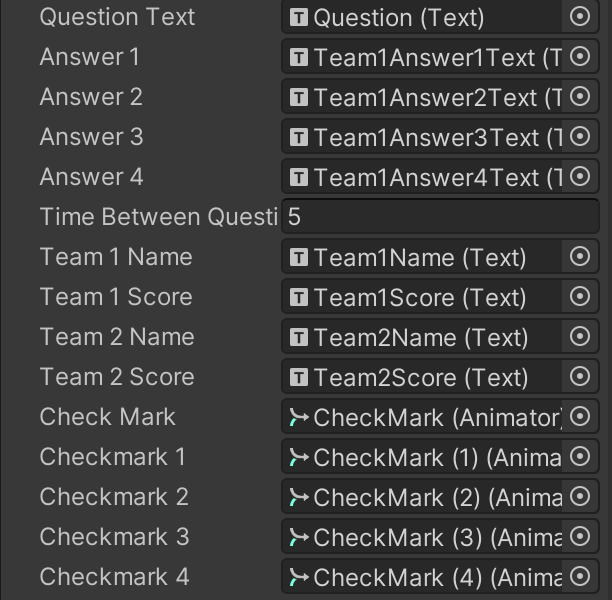
SceneControl
SceneControl execută scriptul SceneControl.cs, apelarea acestuia fiind diferită de GameManager, care rulează automat. Un buton apelează câte o anumită funcție din acest obiect, cu anumiți parametri.
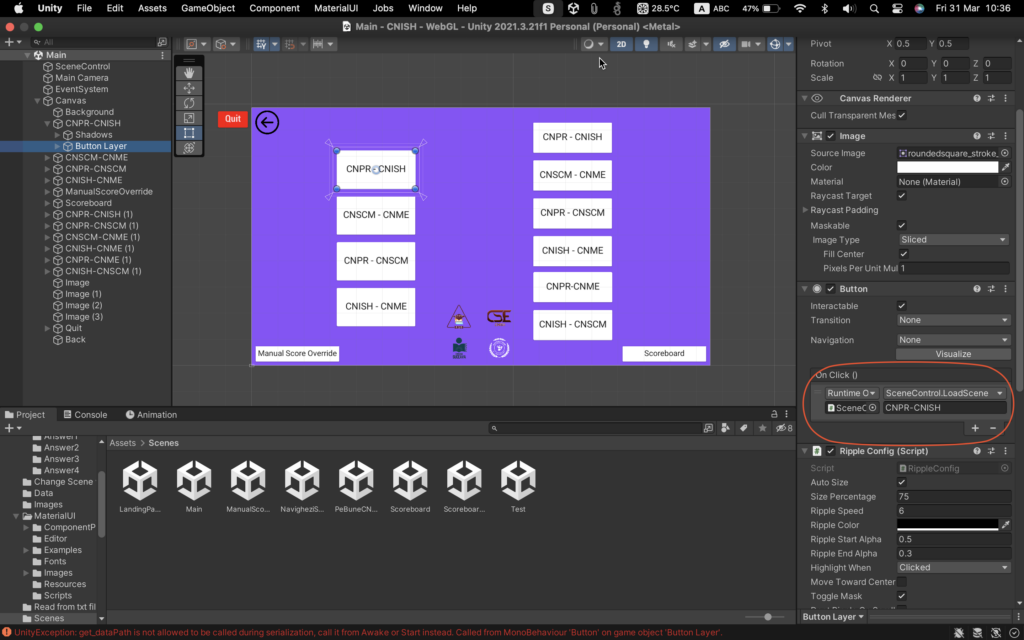
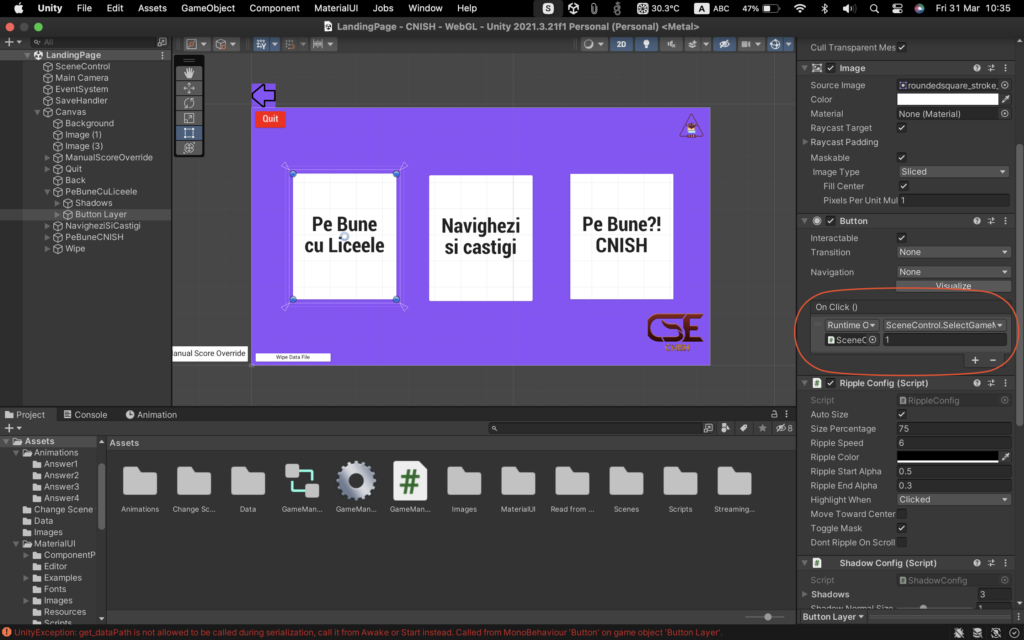